How to create Scalable Programs to be a Developer By Gustavo Woltmann
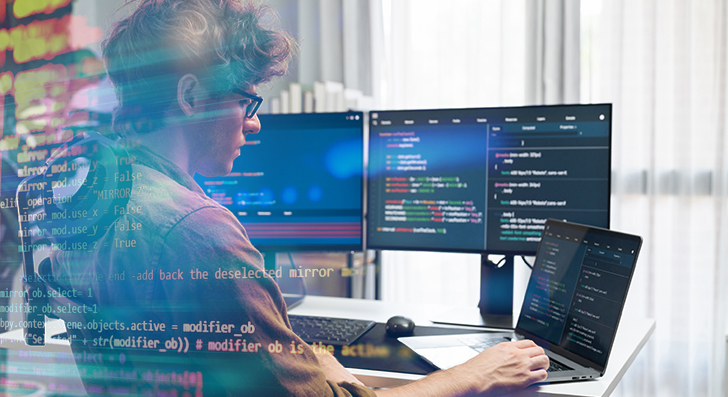
Scalability usually means your application can cope with growth—much more consumers, a lot more knowledge, plus much more traffic—with no breaking. Like a developer, building with scalability in your mind saves time and worry later on. Here’s a transparent and useful guide to assist you to start off by Gustavo Woltmann.
Style for Scalability from the beginning
Scalability is not a little something you bolt on later on—it ought to be element within your program from the start. Several purposes fall short every time they increase fast due to the fact the original layout can’t handle the extra load. To be a developer, you should Imagine early about how your process will behave under pressure.
Start off by creating your architecture to get adaptable. Stay away from monolithic codebases where by every thing is tightly linked. In its place, use modular design and style or microservices. These designs crack your app into more compact, unbiased parts. Just about every module or services can scale on its own devoid of influencing the whole program.
Also, contemplate your databases from working day 1. Will it have to have to handle a million consumers or merely 100? Pick the right sort—relational or NoSQL—determined by how your facts will expand. Prepare for sharding, indexing, and backups early, Even when you don’t have to have them yet.
An additional critical issue is to avoid hardcoding assumptions. Don’t create code that only operates less than current circumstances. Take into consideration what would come about If the person foundation doubled tomorrow. Would your application crash? Would the database slow down?
Use style and design styles that guidance scaling, like information queues or celebration-pushed programs. These support your app manage a lot more requests devoid of receiving overloaded.
If you Create with scalability in mind, you're not just preparing for fulfillment—you happen to be minimizing foreseeable future head aches. A properly-planned method is less complicated to take care of, adapt, and increase. It’s greater to organize early than to rebuild later.
Use the Right Databases
Deciding on the suitable database is really a vital Component of constructing scalable programs. Not all databases are built a similar, and utilizing the Incorrect one can gradual you down as well as result in failures as your application grows.
Start off by knowing your data. Could it be extremely structured, like rows inside of a desk? If Indeed, a relational database like PostgreSQL or MySQL is a great suit. These are solid with relationships, transactions, and regularity. They also aid scaling strategies like go through replicas, indexing, and partitioning to take care of far more traffic and facts.
In case your details is much more adaptable—like user exercise logs, item catalogs, or paperwork—take into account a NoSQL option like MongoDB, Cassandra, or DynamoDB. NoSQL databases are greater at handling massive volumes of unstructured or semi-structured details and may scale horizontally additional effortlessly.
Also, look at your study and publish patterns. Will you be doing a great deal of reads with much less writes? Use caching and read replicas. Have you been managing a heavy compose load? Check into databases that can cope with high compose throughput, as well as party-based info storage programs like Apache Kafka (for non permanent data streams).
It’s also wise to Consider in advance. You may not require Innovative scaling capabilities now, but deciding on a databases that supports them indicates you gained’t want to change later on.
Use indexing to hurry up queries. Avoid pointless joins. Normalize or denormalize your info according to your entry designs. And constantly keep an eye on databases effectiveness while you improve.
Briefly, the right database depends on your app’s composition, velocity requires, And exactly how you be expecting it to improve. Acquire time to choose correctly—it’ll preserve loads of issues later on.
Enhance Code and Queries
Quickly code is key to scalability. As your application grows, each individual smaller hold off adds up. Badly created code or unoptimized queries can decelerate effectiveness and overload your process. That’s why it’s essential to Make successful logic from the start.
Commence by creating thoroughly clean, easy code. Steer clear of repeating logic and remove nearly anything unneeded. Don’t pick the most advanced Alternative if a straightforward one particular performs. Maintain your functions shorter, centered, and straightforward to check. Use profiling instruments to discover bottlenecks—spots in which your code requires as well extensive to operate or employs a lot of memory.
Subsequent, look at your databases queries. These typically sluggish issues down much more than the code alone. Ensure that Each and every query only asks for the info you really require. Avoid Pick out *, which fetches every thing, and in its place pick specific fields. Use indexes to hurry up lookups. And steer clear of accomplishing too many joins, especially across big tables.
For those who recognize a similar details currently being asked for repeatedly, use caching. Shop the final results quickly utilizing instruments like Redis or Memcached so you don’t must repeat highly-priced operations.
Also, batch your databases functions if you can. Instead of updating a row one by one, update them in groups. This cuts down on overhead and would make your application much more productive.
Make sure to exam with large datasets. Code and queries that perform wonderful with one hundred documents may well crash whenever they have to take care of one million.
In short, scalable apps are quick apps. Maintain your code restricted, your queries lean, and use caching when essential. These methods enable your software keep smooth and responsive, even as the load raises.
Leverage Load Balancing and Caching
As your app grows, it has to deal with a lot more consumers and a lot more website traffic. If anything goes as a result of a person server, it will eventually immediately turn into a bottleneck. That’s where load balancing and caching come in. Both of these instruments enable maintain your app quickly, stable, and scalable.
Load balancing spreads incoming traffic throughout many servers. In place of just one server undertaking every one of the get the job done, the load balancer routes end users to diverse servers depending on availability. This means no one server will get overloaded. If a single server goes down, the load balancer can deliver visitors to the Some others. Equipment like Nginx, HAProxy, or cloud-primarily based solutions from AWS and Google Cloud make this very easy to create.
Caching is about storing knowledge temporarily so it might be reused promptly. When consumers request the exact same details again—like an item webpage or a profile—you don’t should fetch it from your databases whenever. You are able to serve it with the cache.
There are 2 popular forms of caching:
1. Server-facet caching (like Redis or Memcached) retailers details in memory for rapidly access.
2. Shopper-side caching (like browser caching or CDN caching) suppliers static information near the consumer.
Caching reduces databases load, increases pace, and will make your app additional effective.
Use caching for things which don’t change typically. And always be sure your cache is current when info does improve.
In brief, load balancing and caching are easy but strong resources. Jointly, they help your app take care of extra consumers, keep speedy, and Recuperate from troubles. If you propose to develop, you may need both of those.
Use Cloud and Container Tools
To make scalable applications, you will need instruments that permit your application grow very easily. That’s the place cloud platforms and containers are available. They offer you flexibility, decrease setup time, and make scaling Considerably smoother.
Cloud platforms like Amazon World-wide-web Products and services (AWS), Google Cloud Platform (GCP), and Microsoft Azure Enable you to lease servers and expert services as you would like them. You don’t have to acquire hardware or guess long run capacity. When visitors boosts, you can include much more assets with just a couple clicks or mechanically applying vehicle-scaling. When website traffic drops, you may scale down to economize.
These platforms also present products and services like managed databases, storage, load balancing, and stability applications. You can focus on building your app instead of managing infrastructure.
Containers are A further critical Resource. A container deals your read more app and every thing it must operate—code, libraries, options—into 1 device. This can make it effortless to move your app between environments, from your notebook on the cloud, without having surprises. Docker is the most popular Software for this.
Once your app uses multiple containers, applications like Kubernetes assist you to manage them. Kubernetes handles deployment, scaling, and Restoration. If a single part within your app crashes, it restarts it immediately.
Containers also make it very easy to independent parts of your application into solutions. You could update or scale areas independently, that is perfect for efficiency and trustworthiness.
In a nutshell, using cloud and container resources usually means it is possible to scale quick, deploy quickly, and Recuperate quickly when complications transpire. If you would like your application to expand without the need of boundaries, start employing these tools early. They help save time, decrease chance, and help you keep focused on constructing, not correcting.
Check Anything
In the event you don’t keep an eye on your software, you won’t know when items go Erroneous. Checking helps you see how your app is accomplishing, spot concerns early, and make greater conclusions as your application grows. It’s a important Section of making scalable techniques.
Start off by monitoring essential metrics like CPU usage, memory, disk space, and response time. These let you know how your servers and companies are accomplishing. Applications like Prometheus, Grafana, Datadog, or New Relic can help you gather and visualize this info.
Don’t just keep an eye on your servers—watch your application far too. Regulate how much time it's going to take for users to load pages, how frequently errors take place, and the place they arise. Logging instruments like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly may help you see what’s occurring within your code.
Create alerts for critical troubles. By way of example, When your response time goes over a limit or a service goes down, you should get notified immediately. This allows you take care of challenges rapid, typically ahead of consumers even detect.
Monitoring can also be helpful whenever you make modifications. If you deploy a completely new element and see a spike in errors or slowdowns, you could roll it again just before it leads to serious problems.
As your app grows, traffic and facts boost. With out checking, you’ll overlook signs of issues right up until it’s as well late. But with the ideal applications in position, you stay on top of things.
In short, checking assists you keep the app responsible and scalable. It’s not nearly recognizing failures—it’s about knowing your system and making certain it really works effectively, even stressed.
Ultimate Views
Scalability isn’t just for major businesses. Even smaller apps will need a strong foundation. By building very carefully, optimizing sensibly, and using the ideal resources, you could Develop applications that grow easily devoid of breaking under pressure. Commence smaller, think huge, and Establish intelligent.